Now on the Next Line Use Console log Again to Log Out the Last Item in the Array
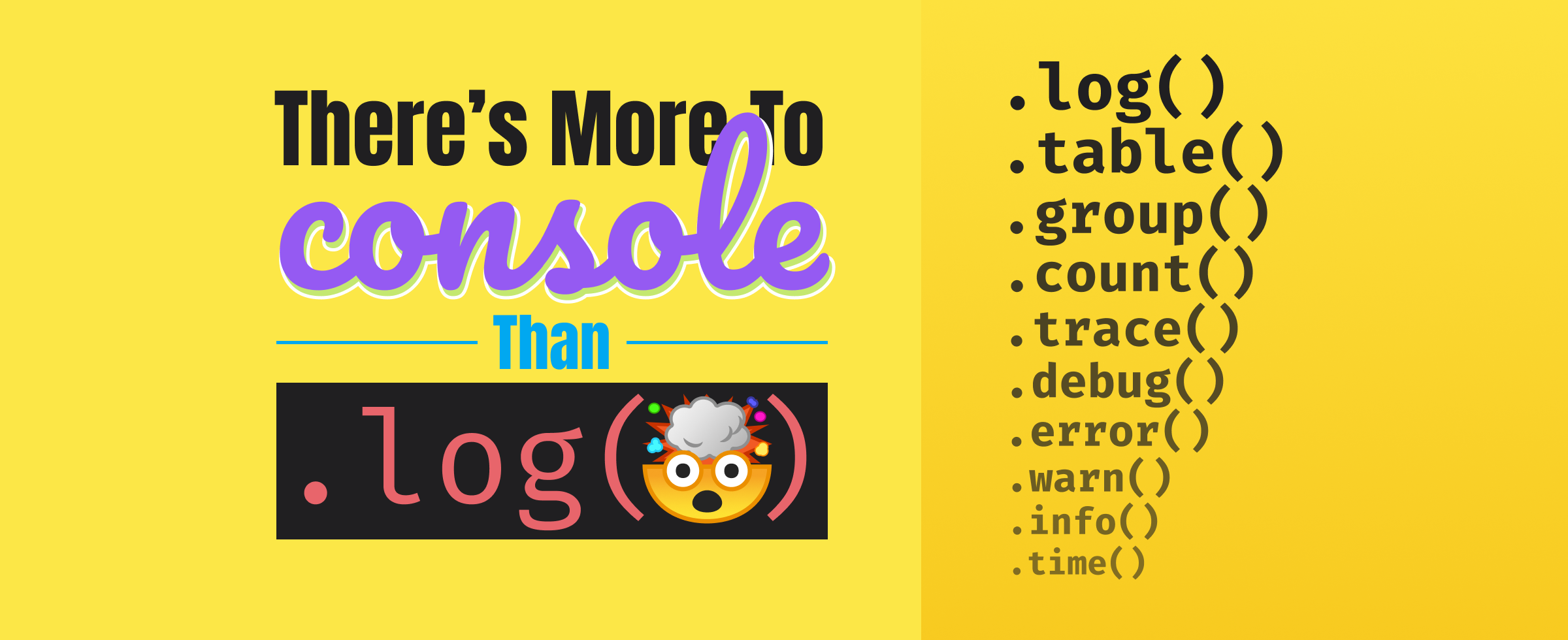
Logging letters to the console is a very basic fashion to diagnose and troubleshoot minor issues in your code.
Simply, did y'all know that there is more to console
than just log
? In this article, I'll show you how to print to the console in JS, as well every bit all of the things you didn't know console
could do.
Firefox Multi-line Editor Console
If you lot've never used the multi-line editor mode in Firefox, you should give it a endeavor right at present!
Just open the console, Ctrl+Shift+Thousand
or F12
, and in the top right you will encounter a button that says "Switch to multi-line editor fashion". Alternatively, you can press Ctrl+B
.
This gives you a multi-line code editor correct inside Firefox.
panel.log
Permit's kickoff with a very basic log example.
permit 10 = ane console.log(x)
Type that into the Firefox panel and run the code. You tin can click the "Run" button or press Ctrl+Enter
.
In this instance, we should run across "i" in the panel. Pretty straightforward, right?
Multiple Values
Did you know that you can include multiple values? Add a cord to the beginning to easily identify what it is yous are logging.
permit x = ane console.log("x:", x)
Just what if we take multiple values that nosotros want to log?
let x = 1 allow y = ii let z = 3
Instead of typing console.log()
iii times we can include them all. And we can add a string before each of them if we wanted, too.
allow x = i let y = two let z = 3 console.log("10:", 10, "y:", y, "z:", z)
But that'south likewise much work. Just wrap them with curly braces! Now yous get an object with the named values.
allow x = one allow y = ii let z = 3 console.log( {x, y, z} )

You can do the same thing with an object.
let user = { name: 'Jesse', contact: { email: 'codestackr@gmail.com' } } panel.log(user) console.log({user})
The first log will print the properties within the user object. The second will place the object equally "user" and impress the backdrop within it.
If you are logging many things to the console, this can help y'all to identify each log.
Variables within the log
Did you know that you can use portions of your log every bit variables?
panel.log("%s is %d years quondam.", "John", 29)
In this case, %s
refers to a string pick included subsequently the initial value. This would refer to "John".
The %d
refers to a digit choice included later on the initial value. This would refer to 29.
The output of this argument would exist: "John is 29 years old.".
Variations of logs
There are a few variations of logs. In that location is the most widely used console.log()
. But there is as well:
console.log('Console Log') panel.info('Console Info') console.debug('Panel Debug') panel.warn('Console Warn') console.error('Console Error')
These variations add styling to our logs in the panel. For case, the warn
will exist colored yellow, and the error
volition be colored red.
Note: The styles vary from browser to browser.
Optional Logs
Nosotros can impress messages to the console conditionally with console.assert()
.
let isItWorking = imitation console.assert(isItWorking, "this is the reason why")
If the outset argument is false, then the message will be logged.
If we were to change isItWorking
to true
, then the message will not be logged.
Counting
Did you know that you lot can count with console?
for(i=0; i<10; i++){ console.count() }
Each iteration of this loop volition print a count to the console. You volition meet "default: one, default: two", and so on until it reaches 10.
If you run this same loop once again you will see that the count picks upward where it left off; eleven - 20.
To reset the counter nosotros can use console.countReset()
.
And, if yous want to name the counter to something other than "default", yous can!
for(i=0; i<10; i++){ console.count('Counter 1') } console.countReset('Counter ane')
Now that we have added a characterization, yous will see "Counter 1, Counter ii", and then on.
And to reset this counter, we accept to pass the name into countReset
. This manner you can have several counters running at the same time and simply reset specific ones.
Rail Time
Likewise counting, you can also time something like a stopwatch.
To first a timer we can use console.time()
. This volition not do annihilation past itself. So, in this example, nosotros will use setTimeout()
to emulate code running. Then, within the timeout, nosotros will stop our timer using console.timeEnd()
.
console.fourth dimension() setTimeout(() => { panel.timeEnd() }, 5000)
As you would look, after 5 seconds, we volition have a timer end log of v seconds.
We tin also log the current fourth dimension of our timer while it's running, without stopping information technology. We exercise this past using console.timeLog()
.
console.fourth dimension() setTimeout(() => { console.timeEnd() }, 5000) setTimeout(() => { console.timeLog() }, 2000)
In this example, we will get our 2 second timeLog
first, then our five second timeEnd
.
Only the same as the counter, nosotros can label timers and accept multiple running at the aforementioned time.
Groups
Another thing that you lot can exercise with log
is group them. ?
We kickoff a group by using console.group()
. And we end a grouping with console.groupEnd()
.
console.log('I am not in a group') console.group() console.log('I am in a group') console.log('I am too in a grouping') console.groupEnd() panel.log('I am not in a group')
This group of logs volition exist collapsible. This makes it like shooting fish in a barrel to identify sets of logs.
By default, the group is not collapsed. Yous can set information technology to collapsed past using console.groupCollapsed()
in place of console.grouping()
.
Labels tin can also be passed into the grouping()
to amend identify them.
Stack Trace
You can likewise do a stack trace with console
. Merely add it into a role.
function one() { two() } function two() { iii() } function iii() { console.trace() } one()
In this example, we have very simple functions that only telephone call each other. So, in the last office, we call panel.trace()
.
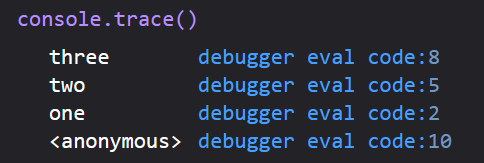
Tables
Here's one of the well-nigh listen-blowing uses for console: panel.tabular array()
.
So let'due south set some information to log:
let devices = [ { name: 'iPhone', brand: 'Apple tree' }, { proper noun: 'Galaxy', brand: 'Samsung' } ]
Now we'll log this information using panel.table(devices)
.
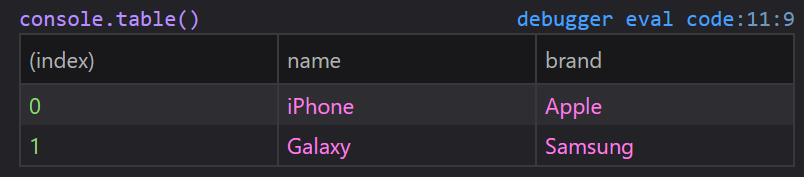
But wait – information technology gets better!
If we only want the brands, merely console.tabular array(devices, ['make'])
!

How about a more complex example? In this instance, we'll use jsonplaceholder.
async function getUsers() { let response = await fetch('https://jsonplaceholder.typicode.com/users') let data = await response.json() console.table(data, ['proper noun', 'email']) } getUsers()
Here nosotros are just printing the "name" and "email". If you console.log
all of the data, yous volition see that at that place are many more backdrop for each user.
Style ?
Did you know that you can use CSS properties to style your logs?
To practice this, we employ %c
to specify that we have styles to add. The styles become passed into the 2nd statement of the log
.
panel.log("%c This is yellow text on a blueish background.", "color:xanthous; background-color:blue")
You tin can use this to make your logs stand out.
Clear
If y'all are trying to troubleshoot an outcome using logs, you lot may be refreshing a lot and your panel may get chaotic.
Just add together console.clear()
to the top of your code and y'all'll have a fresh console every time you lot refresh. ?
Just don't add together it to the bottom of your lawmaking, lol.
Thanks for Reading!
If you want to revisit the concepts in this commodity via video, y'all can check out this video-version I fabricated here.

I'm Jesse from Texas. Check out my other content and let me know how I can help you on your journey to becoming a web developer.
- Subscribe To My YouTube
- Say Hello! Instagram | Twitter
- Sign Upward For My Newsletter
Acquire to code for free. freeCodeCamp's open up source curriculum has helped more than 40,000 people get jobs every bit developers. Get started
Source: https://www.freecodecamp.org/news/javascript-console-log-example-how-to-print-to-the-console-in-js/
0 Response to "Now on the Next Line Use Console log Again to Log Out the Last Item in the Array"
Post a Comment